TLDR; Here is an APK I created wrapping chat.openai.com
Update: The generated APK will not work with Google sign in unless you also update the user-agent to match one Google OAuth accepts. This is left as an exercise to the reader (or you can create an OpenAI account with an email and password).
As we find ourselves interacting with ChatGPT more and more these days, it feels silly that the only way official way on mobile is through a web browser. Although this has many benefits (tabs), it's sometimes nice to have a dedicated app icon and have the app run in standalone mode without the extra browser UI elements. This could all be trivially resolved if OpenAI provided a PWA manifest, but they currently do not. Fortunately, it's really simple to build an iframe wrapper with React Native / Expo.
What better way to start out writing a wrapper, than getting ChatGPT to do it for us!
ChatGPT Expo Start Project Conversation
Me: Can you write a react native (expo) application that simplify iframes chat.openai.com?
As an AI language model, I can provide you with instructions on how to create a bare-bones React Native (Expo) application that can simplify iframes chat.openai.com. Here are the steps:
Install the Expo CLI by running the following command in your terminal:
npm install -g expo-cli
Create a new React Native project using the Expo CLI by running the following command:
expo init chat-app
This will create a new directory called chat-app with all the necessary files and dependencies to get started.
Open the project directory in your preferred code editor and navigate to the App.js file. Replace the existing code with the following:
import React from 'react'; import { WebView } from 'react-native-webview'; export default function App() { return ( <WebView source={{ uri: 'https://chat.openai.com/' }} style={{ flex: 1 }} /> ); }
This code imports the WebView component from react-native-webview and uses it to render the chat.openai.com website inside the app.
Save the file and run the app using the following command:
expo start
This will start the Expo development server and open a web page in your browser with a QR code.
Install the Expo Go app on your mobile device and scan the QR code with your camera to open the app on your device.
That's it! You should now see the chat.openai.com website rendered inside your React Native app. This is a very bare-bones implementation, but you can customize the styling and functionality of the app as needed.
Using the above instructions (and updating slightly for 2023, using npx create-expo-app <appname>
), we have a basic running Expo app that iframes ChatGPT. The only thing that is slightly lacking is the status bar on Android showing the iframe UI behind it, making the text hard to read and UI hard to click. For this, we can change the translucent
option to false, and give the status bar a background color. We can also set it to light
so the status bar text renders in white.
const App = () => {
return <>
<WebView
source={{ uri: 'https://chat.openai.com/' }}
style={{ flex: 1 }}
/>
<StatusBar style="light" backgroundColor="#343541" translucent={false} />
</>;
};
From here, the steps to a standalone applications are pretty simple.
- Follow the guide to create the icons files using the Figma template. Just drop in a ChatGPT SVG and it should look like this.
- Add a build config to your app.json so that an apk is generated in the build process
"build": {
"preview": {
"android": {
"buildType": "apk"
}
}
},
- Generate a build using your expo eas build account (create one here). Once you have an account, install
eas-cli
withnpm install -g eas-cli
. Then logineas login
. Then create a buildeas build -p android -e preview
. - Once the build completes, you'll get a URL to download the build, you can open this APK file on your Android device and install it.
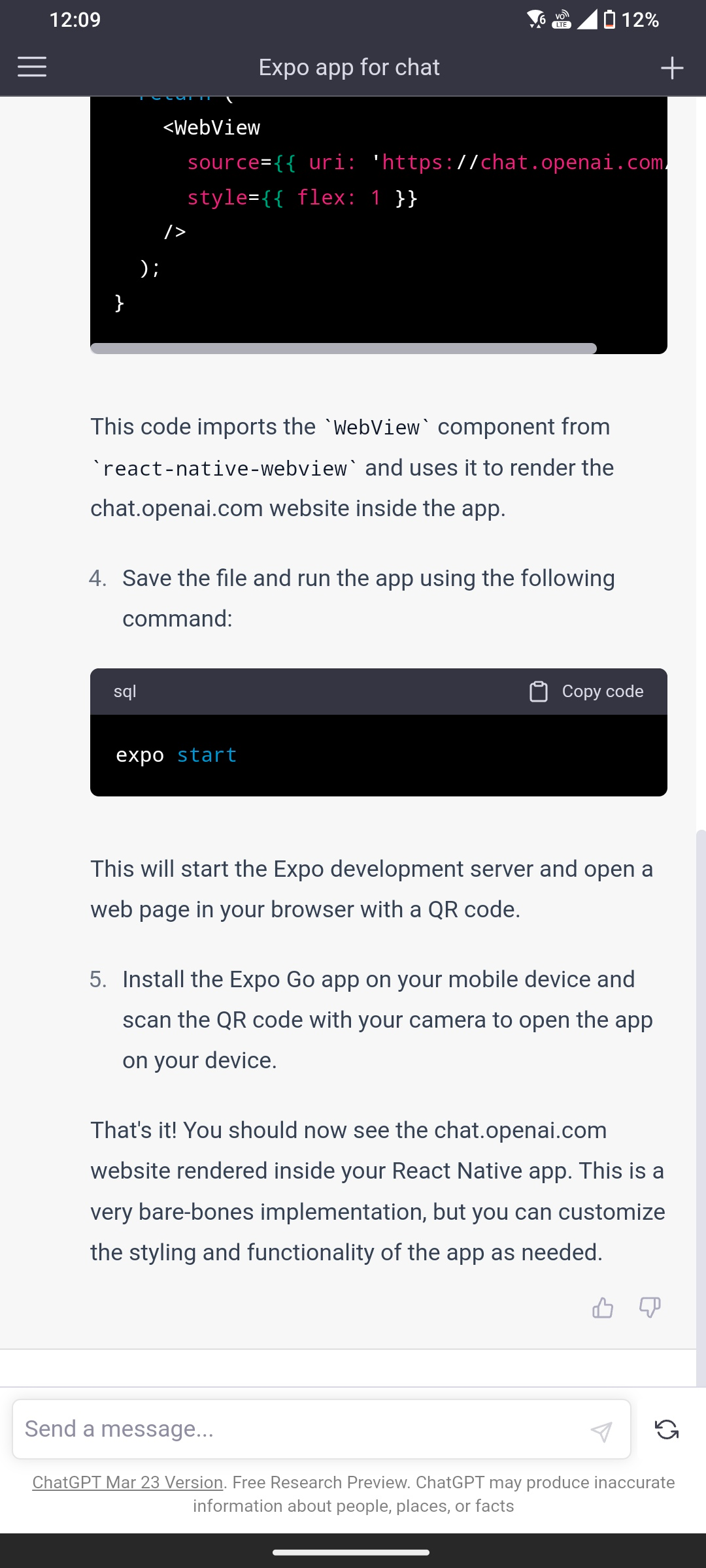
That's it! You've created an Android application that iframes ChatGPT to install on your own device and run in standalone mode. Full final source code of the application can be seen here. If you just want to download a pre-built APK, click below:
Download Chat GPT - React Native Shell APK